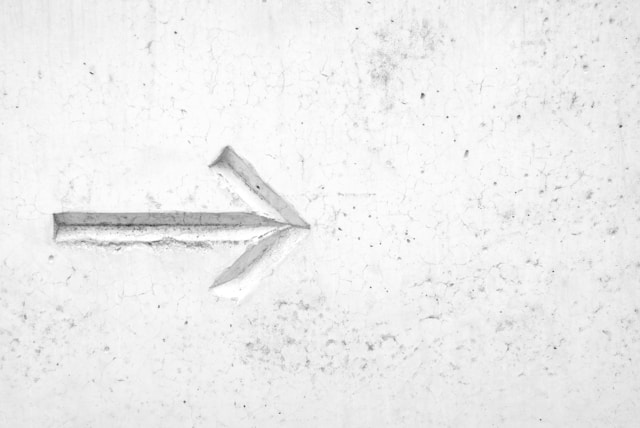
Cypress provides you with the below hooks
describe('Hooks', () => {
before(() => {
// runs once before all tests in the block
})
beforeEach(() => {
// runs before each test in the block
})
afterEach(() => {
// runs after each test in the block
})
after(() => {
// runs once after all tests in the block
})
})
Note that there is no way to run an after test hook (for a specific test case).
There is afterEach() — which runs after every test.
and there is after() — which runs at the end.
Why is this even required?
There are some use cases for this. Also if it wouldn’t then why would some popular framework’s in other languages even have it.
Let’s take an example use case!
Let us assume that during testing you are using / sharing one entity for testing multiple test cases and after each test you need to reset the state back to the original state but the code / procedure to reset the state from current state to original state is distinctly different in each case.
How to implement this in Cy.
Let us start by creating a custom command in command.js file as below.
describe('Sample test suite', () => {
afterEach(() => {
// runs after each test case
cy.get('@testTeardownId').then(id => {
if (id === 'tc1') {
cy.log('Running test teardown for first test')
}
else if (id === 'tc2') {
cy.log('Running test teardown for second test')
}
})
})
it("Sample test case 1", () => {
cy.setTestTeardownId('tc1')
cy.log('Test Case 1')
})
it("Sample test case 2", () => {
cy.setTestTeardownId('tc2')
cy.log('Test Case 2')
})
})
Your execution will look something like this
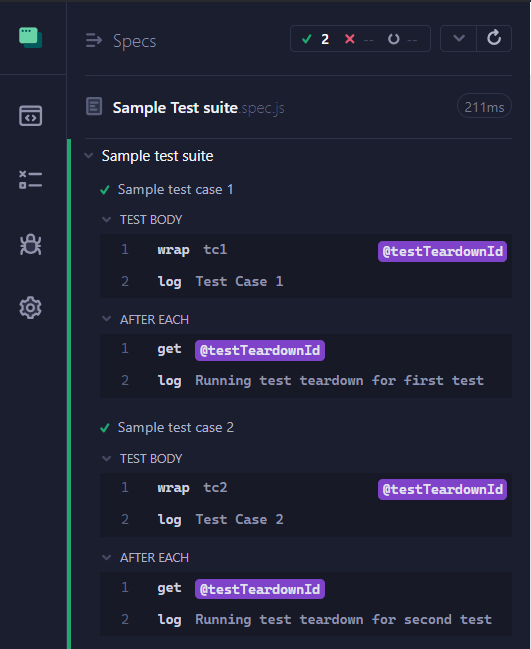
We can see that we can now have test case specific teardown code written.
This is sometimes important because we want to make sure teardown (crucial to maintain test environment hygiene) run’s irrespective of what happens to the test (passed or failed).
Bonus Tip — Always make sure you are using more reliable API calls such as REST API, DB Queries etc. in teardown’s / other hooks and DO NOT use UI to do the same due to the simple fact that UI actions are less reliable and time consuming.
p.s. — There is no use case for a before a specific test hook. Perhaps just put some API calls before your actual test begins in the test block.